ADOBE EXPERIENCE MANAGER (AEM)
Stubbing Data with AEM JavaScript Use-API
An Adobe Experience Manager (AEM) example of a new blank slate starter component with stub data. The JavaScript Use-API returns the data to the HTL template for development.
Features
The project includes a basic helloworld
component that we will copy to create the new component. Our example will be titled “Products Component”. Read this post for help on creating an AEM Maven Project with the archetype.
cd ui.apps/src/main/content/jcr_root/apps/myproject/components/content
cp -R helloworld/ products
mv products/helloworld.html products/products.html
Update the jcr:title
property in features/.content.xml
replacing =“Hello World Component”`. e.g.,
<?xml version="1.0" encoding="UTF-8"?>
<jcr:root xmlns:cq="http://www.day.com/jcr/cq/1.0" xmlns:jcr="http://www.jcp.org/jcr/1.0"
jcr:primaryType="cq:Component"
jcr:title="Products Component"
componentGroup="myproject.Content"/>
In products/products.html
, comment out the pre
element and it’s content.
<!--/*
<pre data-sly-use.hello="com.adobe.aem.dev.myproject.core.models.HelloWorldModel">
HelloWorldModel says:
${hello.message}
</pre>
*/-->
Deploy the new products
component folder to AEM. This can be accomplished in a variety of ways. For example, with deployments such as this, that do not require a Maven build, you can use the AEM repo tool, FileVault VLT or Eclipse to name a few. read more ….
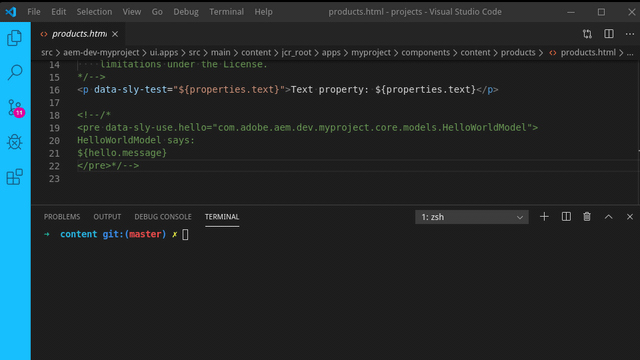
Stub Data
This sample bakery data from opensource.adobe.com gives us a nice example we can use for our data source.
products.js
use(function() {
let products = [];
products = [
{
"id": "0001",
"type": "donut",
"name": "Cake",
"ppu": 0.55,
"batters":
{
"batter":
[
{ "id": "1001", "type": "Regular" },
{ "id": "1002", "type": "Chocolate" },
{ "id": "1003", "type": "Blueberry" },
{ "id": "1004", "type": "Devil's Food" }
]
},
"topping":
[
{ "id": "5001", "type": "None" },
{ "id": "5002", "type": "Glazed" },
{ "id": "5005", "type": "Sugar" },
{ "id": "5007", "type": "Powdered Sugar" },
{ "id": "5006", "type": "Chocolate with Sprinkles" },
{ "id": "5003", "type": "Chocolate" },
{ "id": "5004", "type": "Maple" }
]
},
{
"id": "0002",
"type": "donut",
"name": "Raised",
"ppu": 0.55,
"batters":
{
"batter":
[
{ "id": "1001", "type": "Regular" }
]
},
"topping":
[
{ "id": "5001", "type": "None" },
{ "id": "5002", "type": "Glazed" },
{ "id": "5005", "type": "Sugar" },
{ "id": "5003", "type": "Chocolate" },
{ "id": "5004", "type": "Maple" }
]
},
{
"id": "0003",
"type": "donut",
"name": "Old Fashioned",
"ppu": 0.55,
"batters":
{
"batter":
[
{ "id": "1001", "type": "Regular" },
{ "id": "1002", "type": "Chocolate" }
]
},
"topping":
[
{ "id": "5001", "type": "None" },
{ "id": "5002", "type": "Glazed" },
{ "id": "5003", "type": "Chocolate" },
{ "id": "5004", "type": "Maple" }
]
},
{
"id": "0004",
"type": "bar",
"name": "Bar",
"ppu": 0.75,
"batters":
{
"batter":
[
{ "id": "1001", "type": "Regular" },
]
},
"topping":
[
{ "id": "5003", "type": "Chocolate" },
{ "id": "5004", "type": "Maple" }
],
"fillings":
{
"filling":
[
{ "id": "7001", "name": "None", "addcost": 0 },
{ "id": "7002", "name": "Custard", "addcost": 0.25 },
{ "id": "7003", "name": "Whipped Cream", "addcost": 0.25 }
]
}
},
{
"id": "0005",
"type": "twist",
"name": "Twist",
"ppu": 0.65,
"batters":
{
"batter":
[
{ "id": "1001", "type": "Regular" },
]
},
"topping":
[
{ "id": "5002", "type": "Glazed" },
{ "id": "5005", "type": "Sugar" },
]
},
{
"id": "0006",
"type": "filled",
"name": "Filled",
"ppu": 0.75,
"batters":
{
"batter":
[
{ "id": "1001", "type": "Regular" },
]
},
"topping":
[
{ "id": "5002", "type": "Glazed" },
{ "id": "5007", "type": "Powdered Sugar" },
{ "id": "5003", "type": "Chocolate" },
{ "id": "5004", "type": "Maple" }
],
"fillings":
{
"filling":
[
{ "id": "7002", "name": "Custard", "addcost": 0 },
{ "id": "7003", "name": "Whipped Cream", "addcost": 0 },
{ "id": "7004", "name": "Strawberry Jelly", "addcost": 0 },
{ "id": "7005", "name": "Rasberry Jelly", "addcost": 0 }
]
}
}
]
return {
products: products
};
});
products.html
<ul data-sly-use.data="data/products.js"
data-sly-list="${data.products}">
<li>${item.name} ${item.type}
<ul data-sly-test="${item.batters.batter}">
<li>Batters
<ul data-sly-list="${item.batters.batter}">
<li>${item.type}</li>
</ul>
</li>
</ul>
<ul data-sly-test="${item.topping}">
<li>Toppings
<ul data-sly-list="${item.topping}">
<li>${item.type}</li>
</ul>
</li>
</ul>
<ul data-sly-test="${item.fillings.filling}">
<li>Fillings
<ul data-sly-list="${item.fillings.filling}">
<li>${item.name}</li>
</ul>
</li>
</ul>
</li>
</ul>
Source Code
Resources
Part 4 of 4 in the AEM JavaScript Use-API series.