Adobe Experience Manager (AEM) Maven Project Part II
An Adobe Experience Manager (AEM) example to demonstrate the Webpack frontend build process included with Maven AEM Project Archetype 22. Follow the steps in part 1 of this series beforehand making sure to include the -DoptionIncludeFrontendModule=general
option when running mvn archetype:generate
. e.g.,
mvn archetype:generate \
-Padobe-public \
-DarchetypeGroupId=com.adobe.granite.archetypes \
-DarchetypeArtifactId=aem-project-archetype \
-DoptionIncludeFrontendModule=general \
-DarchetypeVersion=22
Frontend Build
Navigate to the ui.frontend
folder with you terminal and kickoff the build for the first time to make sure it will run. e.g.,
cd aem-dev-myproject/ui.frontend
npm run start
Note that we did not need to run
npm i
to install the node modules. This was all handled by the archetype during the mvn clean install.
I received the following error:
Node Sass could not find a binding for your current environment: Linux 64-bit with Node.js 8.x
Found bindings for the following environments:
- Linux 64-bit with Node.js 10.x
This usually happens because your environment has changed since running `npm install`.
Run `npm rebuild node-sass` to download the binding for your current environment.
Inspecting, the mvn
build output, node and npm are installed into the projects ui.frontend
folder.
[INFO] --- frontend-maven-plugin:1.7.6:install-node-and-npm (install node and npm) @ aem-dev-myproject.ui.frontend
[INFO] Installing npm version 6.9.0
[INFO] Installing node version v10.13.0
The issue is due to my globally installed node major version that is different from what was installed locally with mvn clean install
.
So I cancelled the process, Ctrl+C and ran npm rebuild node-sass
as instructed.
Then I kicked off the build again, 🎩 wallah, it ran without any errors. The static HTML template should be available at localhost:8080 or the next available port. For more information, see the ui.frontend/README.md
The
node
andnpm
versions used in the maven build are configured in theaem-dev-myproject/pom.xml
for thefrontend-maven-plugin
.
Now let’s see how the frontend build process works using the static HTML template.
Content Page Template
We’re going to modify the footer which we can get the markup for from the content page.
In AEM, under Navigation, select Sites. Then select myproject > us > en and open en for editing. Under Page information, select View as Published. Or load it directly using a URL that has the ?wcmmode=disabled
query string to View as Published. e.g., http://localhost:4502/content/myproject/us/en.html?wcmmode=disabled
If you inspect the footer of the project Content page, you will notice that the experience fragment doesn’t have a CSS class or attribute that identifies it as a footer. We will need a way to select it and apply our CSS rules. The easiect way to do that is by adding a class.
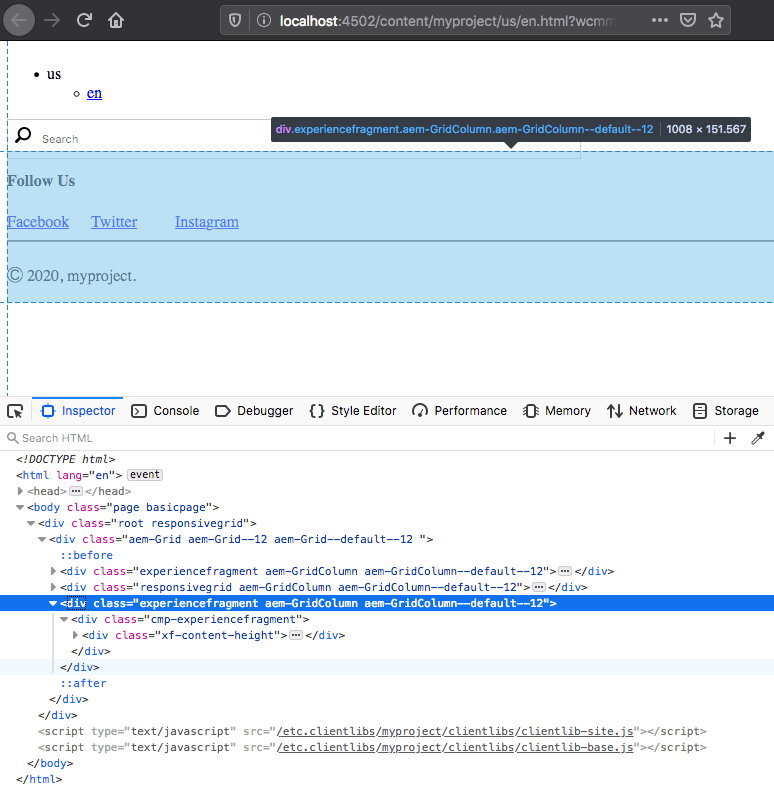
Adding a CSS Class
From the AEM start page, select Tools > Templates. Then select the myproject folder tile, and Edit the Content Page Structure. Select the footer Experience Fragment and its Policy editor from the action bar.
To add a new default CSS class to the footer Experience Fragment, select the myproject Experience Fragment - Footer
policy. Add a Policy Title and a Default CSS class named cmp-experiencefragment--footer
.
Verify CSS Class
Go back to the Content page and View as Published. e.g., http://localhost:4502/content/myproject/us/en.html?wcmmode=disabled to verify that the new cmp-experiencefragment--footer
class is there.
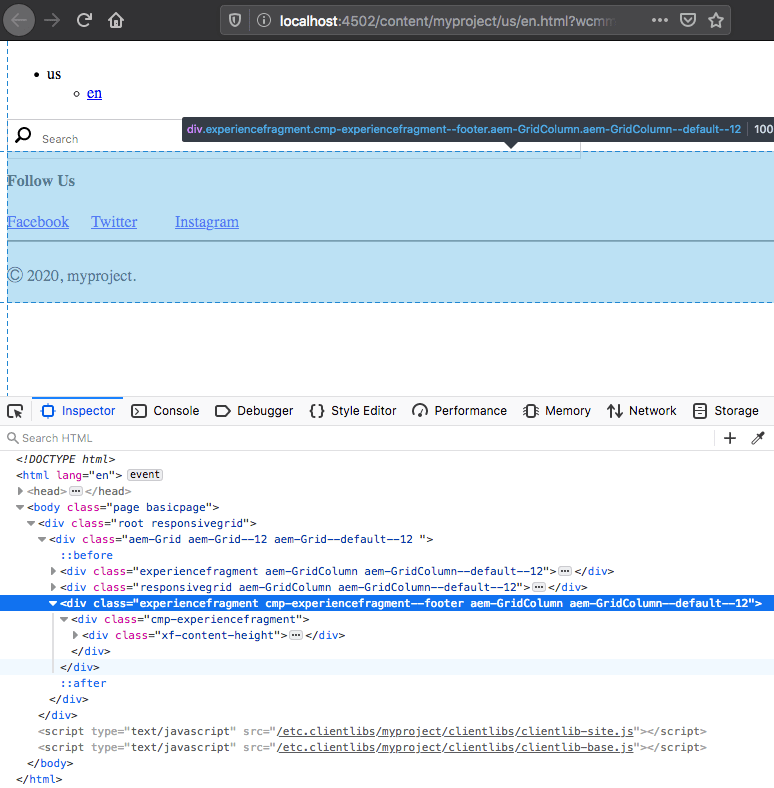
Transfer Configuration Changes for Source Control
Now would be a good time to transfer the configuration changes we made from AEM to your local file system for source control. For this process, we’re going to use the AEM Repo Tool.
To determine which folders under the jcr_root
we need to get
from AEM, use the repo serverdiff
command. Navigate to the ui.content configuration directory for myproject
. e.g.,
cd aem-dev-myproject/ui.content/src/main/content/jcr_root/conf/myproject
Run the repo serverdiff
command to show differences done on the server compared to local for the current directory, myproject
.
repo serverdiff
By inspecting the output for all instances of +++ REMOTE/conf/myproject
, we can determine that these conf/myproject
folders contain updates.
settings/wcm/policies
settings/wcm/template-types
settings/wcm/templates
Run repo get
to import from AEM to your local file system. e.g.,
repo get settings/wcm/policies
repo get settings/wcm/template-types
repo get settings/wcm/templates
If you’re using Visual Studio Code, you can configure terminal tasks to execute repo commands for the active file or it’s folder.
Footer Markup
For the frontend devlopment, we’re going to use Webpack. First thing we need to do is copy the markup we want to develop into our static HTML template. View source of the published view Content page and copy all of the source markup. With your code editor, open the static HTML template at aem-dev-myproject/ui.frontend/src/main/webpack/static/index.html
replacing the contents with the source we copied from the published view of the Content page.
Instead of manually copy and pasting, use
wget
to download the page and output the document as our static HTML template:
cd aem-dev-myproject/ui.frontend
wget --user=admin --password=admin -O src/main/webpack/static/index.html http://localhost:4502/content/myproject/us/en.html?wcmmode=disabled
If you do not want to have the password saved in your command history, replace --password=admin
with --ask-password
.
Like we did at the start of this tutorial, navigate to the ui.frontend
folder and run the build. e.g.,
cd aem-dev-myproject/ui.frontend
npm run start
Make some style changes to the footer, for example, edit the ui.frontend/src/main/webpack/components/content/experiencefragment/scss/experiencefragment.scss
as follows:
experiencefragment.scss
.cmp-experiencefragment--footer {
background: #edf0f5;
}
When you save the file, the Webpack develpment process is watching for the change and reloads the static HTML template. You should be able to see your Sass changes transpiled to CSS in the static HTML template instantly. When you’re ready to stop developing, Ctrl+C to exit the process.
Prepare the ui.frontend
changes for deployment into AEM using one of the following:
npm run dev
- builds client libraries with minification, etc. disabled and source maps enabled.npm run prod
- builds client libraries with minification and optimization (tree shaking, etc), and source maps disabled.
Details are available in the ui.frontend/README.md.
Install
You can install the updated client libraries using the repo tool or run the Maven build to install onto AEM. e.g.,
Option 1, repo tool
cd aem-dev-myproject/ui.apps/src/main/content/jcr_root/apps/myproject/clientlibs
repo put
Option 2, Maven build
cd aem-dev-myproject
mvn clean install -PautoInstallSinglePackage
You can also speed things up by skipping the tests. e.g.,
mvn clean install -PautoInstallSinglePackage -DskipTests
Reload the Content page in AEM to verify.
Additionally, inspect the localhost:4502/etc.clientlibs/myproject/clientlibs/clientlib-site.css file for the updated .cmp-experiencefragment--footer
css rule. The css file is located in ui.apps/src/main/content/jcr_root/apps/myproject/clientlibs/clientlib-site/css/site.css
and is accessible at /etc.clientlibs
using the Proxy Client Libraries Servlet. Read Using Client-Side Libraries for more information.
The allowedProxy
property is set to true on the apps/myproject/clientlibs/clientlib-site
folder to expose it via /etc.clientlibs/clientlib-site
.
That’s it for now. Happy coding!
Source Code
Part 2 of 4 in the AEM Maven Project series.
Adobe Experience Manager (AEM) Maven Project | Adobe Experience Manager (AEM) Maven Project Archetype 23