Below you will find pages that utilize the taxonomy term “JavaScript”
Golf Scorecard React App GIR
React Golf Scorecard application updated to show Greens in Regulation (GIR). For this we needed figure out how to trigger an on change event when the input value for GIR is updated dynamically when strokes and putts are entered. It is recommended that you read Part 1 which contains the information on how to install and build this application locally.
You can view a demo of the app here
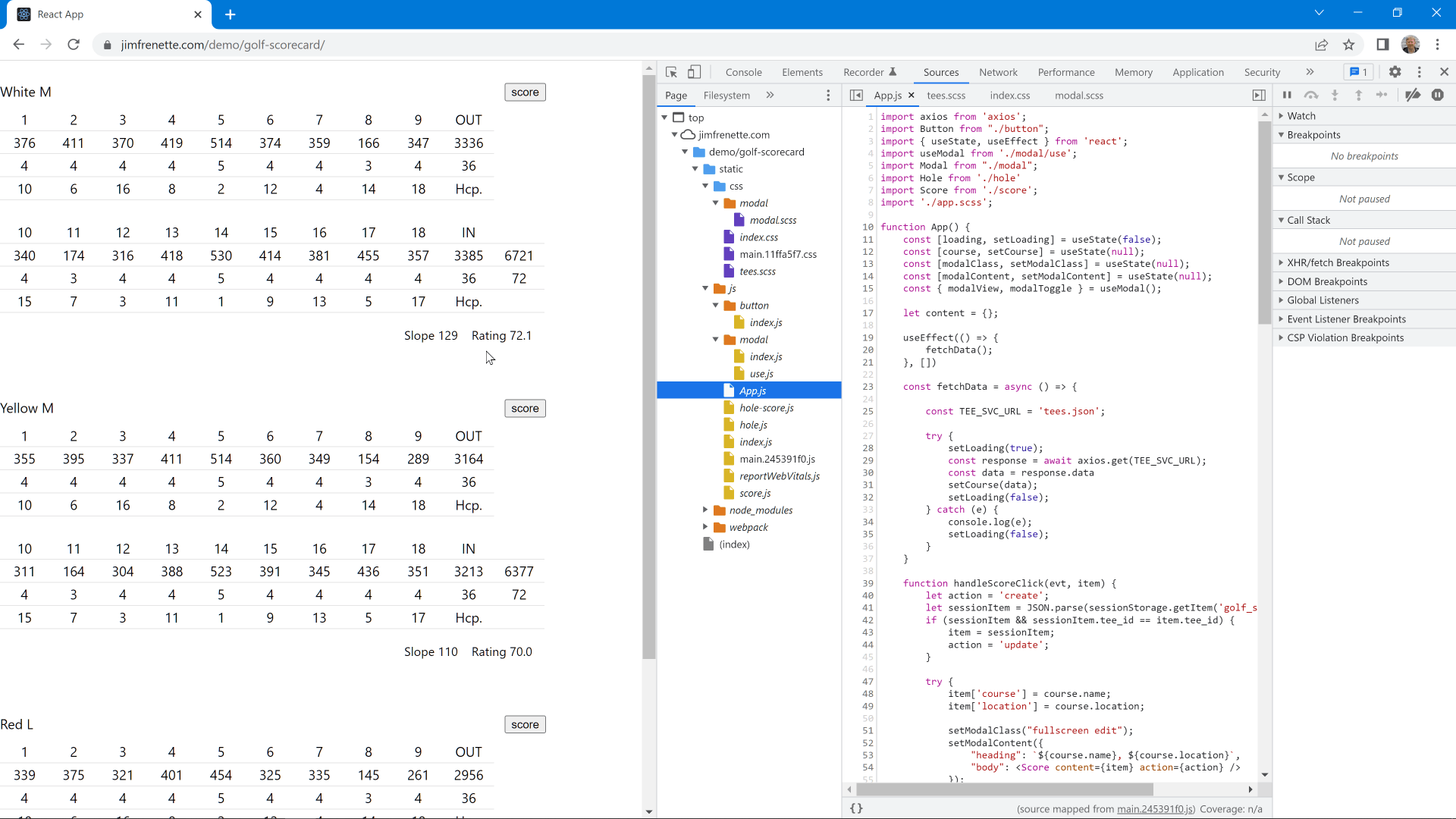
Golf Scorecard React App
This tutorial demonstrates how to create a React application that is used in a great many golf apps, a way to display and edit golf course scorecard data. You can view a demo of the app here
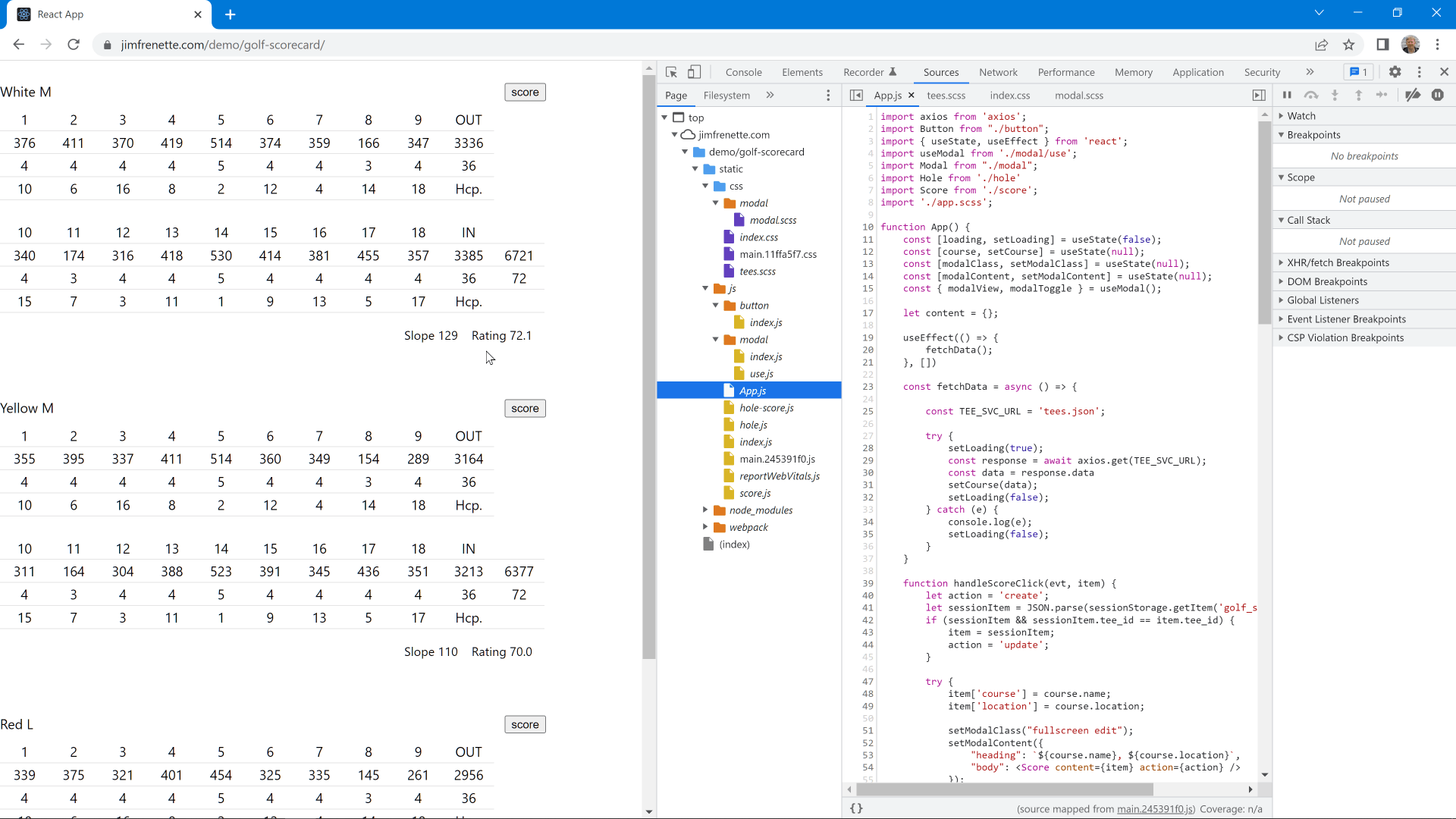
React
Vue.js
querySelector
Basic querySelector
examples including iteration of DOM NodeList returned by querySelectorAll
using either Array.from
or [].slice.call
.
Slick Carousel Responsive slidesToShow Recipe
Designs typically require more than the out of the box demos provide, which is understandable. The slick carousel demos will get you most of the way there and provide examples for various settings. This post is an example of how to dynamically update the slick slidesToShow
value as the viewport is resized, demo.
How To Create a WordPress Shortcode for flickr Albums
How to create a WordPress custom shortcode to display photos from flickr. This post documents using the Slick Lazy Load Photo Grid along with the flickr API to render photo albums in Wordpress Posts wherever the shortcode is entered. Webpack 4, autoprefixer, and babel are included for building the JS and CSS.
CSS Flexbox with Sidebars Toggle
This post documents how to create a fully responsive flexbox content layout that contains a pure CSS open/close toggle for the left and right sidebars. The CSS is built from Sass using Webpack with Autoprefixer. For development, a Webpack Dev Server is included.
Webpack 3 Sass cssnano Autoprefixer Workflow II - Page 2
Webpack 3 Sass cssnano Autoprefixer Workflow II
Slick Lazy Load Photo Grid Using Webpack 3
How to layout and lazy load images in a flexible grid similar to how facebook displays them in a post. Selected images open a lightbox for previewing within a carousel. Image alt text is converted into a caption below the image. YouTube Video
Google Maps API with Webpack
Google Map application that uses a draggable location marker to set address, longitude and latitude geocode inputs. This post covers some of the Webpack tools for both a local development environment and production build of the app constructed of a few JavaScript modules and css.
Laravel User Authentication with Ajax Validation
This post documents how to add Ajax form validation to Laravel User Authentication that is generated by the Artisan console.
Design Patterns
Design patterns are reusable solutions to commonly occurring problems in software design.
Google Maps API with Browserify
This post documents how to use local JavaScript modules with Browserify and the Google Maps JavaScript API. In this example, the Google Map contains a marker that can be dragged to reset the browser form with the marker position location data. Additionally, Browserify shim is used to require jQuery since it is already being loaded from a CDN.
Javascript Frameworks
After discovering yet another interesting Javascript framework recently for web application development (Vue.js), I decided to put together this short list of some the emerging and more popular frameworks out there today for building user interfaces.
Google Maps API RequireJS Module
This post documents how to create a RequireJS module that loads the Google Maps JavaScript API and is used by a web page to populate form inputs with location data. The Google Map contains a marker that can be dragged to reset the form with the marker position location data.
Browserify with Sourcemaps
Browserify lets you write modular JavaScript in node.js style. At the beginning of each module you write, the respective dependencies are added using require statements. Then Browserify compiles the modules along with all of the dependencies into an optimized JavaScript file for use in the browser.
AngularJS v2: Angular.io
At ng-conf 2015 last month it was announced that AngularJS 1.X will continue to reside at angularjs.org and Angular 2.0 will be hosted at angular.io. The new version of Angular is not a major update, it is a complete rewrite. In February, Brad Green announced that Angular 2 was officially Alpha in this First look at App Development in Angular2 video.
JavaScript Templating Options
Javascript templating is a technique to render JSON data in HTML markup with JavaScript.
Wordpress Theme Javascript Optimization
This post shows how to combine and minify multiple javascript files in a Wordpress theme into one javascript file. The benefit is a single request to a javascript file that has been compressed by minification instead of multiple request to larger javascript files.
JavaScript Helper Functions
Various helper functions are included on this page. See DOM Helpers to look for document object helpers.
Grunt JavaScript Task Runner
This tutorial describes how to setup and use the Grunt JavaScript task runner to automate repetitive tasks such as minification and compilation. Grunt is installed using npm, the Node.js package manager. You will also need Git to work with the tagged source code. This makes it easy to reset and compare your working copy of the code at each step. I discovered commit tags while using the AngularJS tutorial.
JavaScript
JavaScript Document Object (DOM) Helpers
The Document object represents the root node of the HTML document. The nodes are organized in a tree structure, called the DOM tree. Objects in the DOM tree may be addressed and manipulated by using methods on the objects.
DotNetNuke CSS and JS Module Development - Part One
During module development, to see all of the js and css files in firebug or your favorite client debugging tool of choice, turn off the file combination provider. In this section at or near the bottom of your web.config, set the provider enableCompositeFiles attributes from true to false as shown in the example below.
ISO Date Time
Useful JavaScript for converting an iso DateTime string into a JavaScript Date object. The Date.prototype.setISO8601 function is from dansnetwork.com/javascript-iso8601rfc3339-date-parser/